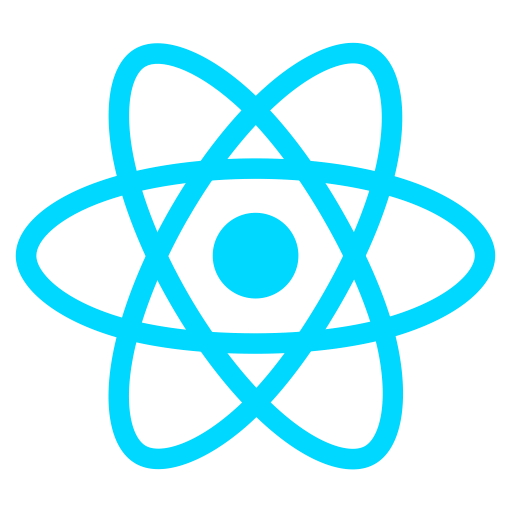
React JS
# Routing using react-router-dom
We can implement routing in a React Web Application using react-router-dom
. So the first thing we have to do is install the package.
If you are using yarn:
yarn add react-router-dom
or using npm:
npm install react-router-dom
In the react-router-dom
we will mainly use these components:
<BrowserRouter/>
- The router that keeps the UI in sync with the URL uses HTML5 History API.<Route/>
- Renders a UI component depending on the URL.<Switch/>
- Renders the first child<Route>
that matches. A<Route>
with no path always matches.<Link>
- Renders a navigation link in HTML.
Let's say we have a Home
page and a About
page for example and we want to route access them at /
and /about
respectively. Also for the routes which are not defined we will throw a custom 404 page - NotFound
.
Assuming we have created our three componenst Home
, About
& NotFound
, let's import them as well as BrowserRouter
, Route
& Switch
from react-router-dom
in our App.js file and setup our routes.
import React, { Component } from 'react'
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom'
import Home from './components/Home.js'
import About from './components/About.js'
import NotFound from './components/NotFound.js'
import './App.css';
class App extends Component {
render() {
return (
<Router>
<Switch>
<Route exact path="/" component={Home}/>
<Route exact path="/about" component={About}/>
<Route component={NotFound}/>
</Switch>
</Router>
);
}
}
export default App;
So here we have used an alias for BrowserRouter
in the import so we can use it simply as Router
in our application. Firstly we will wrap our whole application inside <Router/>
and then using <Switch/>
we have defined the indivisual routes with path
as the route we want to access that component at and exact
means that it should be a complete match.
So we accordingly define our custom routes and the related components. Notice that at the end for the NotFound
component route we haven't specified a path which means that if any route dont match the paths which are defined then it will load the NotFound
component by default.
Therefore we have implemented routing using react-router-dom
.
Now if you wish to navigate to a route in any part of your JSX, we can do so by using <Link/>
.
Firsly we will import it from react-router-dom
import { Link } from 'react-router-dom'
and inside JSX we can use it as:
<Link to="/about">About</Link>
so when we will click on About in the UI it will take us to /about
.
Also to programmatically change route inside any component we can use the props
as:
this.props.history.push('/about')
This is how using react-router-dom
we can implement routing in our application.
Learn More
- Routing using react-router-dom